On this submit I’ll present you find out how to customise Woocommerce orders web page with the assistance of easy code snippets. Now, so as to make this work add your chosen snippets proven to your baby theme’s features.php file or higher but, use a snippet supervisor like Code Snippets.
Video: The right way to Customise Woocommerce Orders web page?
In case you could have a tough time utilizing or following snippets proven beneath, check out the video. In it I’ll present you find out how to use them.
The right way to add an Woocommerce order quantity search subject to the WP Admin prime bar?
For the time being you need to go to the Woocommerce >> Orders web page so as to search orders. With the assistance of this hack you are able to do it straight out of your WordPress admin bar (see screenshot)

add_action('wp_before_admin_bar_render', operate() {
if(!current_user_can('administrator')) {
return;
}
international $wp_admin_bar;
$search_query = '';
if (!empty($_GET['post_type']) && $_GET['post_type'] == 'shop_order' ) {
$search_query = !empty($_GET['s']) ? $_GET['s'] : '';
if($search_query) {
$order = get_post(intval($search_query));
if($order) {
wp_redirect(get_edit_post_link($order->ID, ''));
exit;
}
}
}
$wp_admin_bar->add_menu(array(
'id' => 'admin_bar_shop_order_form',
'title' => '<type methodology="get" motion="'.get_site_url().'/wp-admin/edit.php?post_type=shop_order">
<enter title="s" sort="textual content" placeholder="Order ID" worth="' . esc_attr($search_query) . '" type="width:100px; top: 25px; padding-left: 5px;">
<button class="button button-primary" type="top: 25px; padding: 0px 10px 0px 10px; margin-left: -5px;">Verify this order</button>
<enter title="post_type" worth="shop_order" sort="hidden">
// Add an Woocommerce order quantity search subject to the WP Admin prime bar
add_action('wp_before_admin_bar_render', operate() {
if(!current_user_can('administrator')) {
return;
}
international $wp_admin_bar;
$search_query = '';
if (!empty($_GET['post_type']) && $_GET['post_type'] == 'shop_order' ) {
$search_query = !empty($_GET['s']) ? $_GET['s'] : '';
if($search_query) {
$order = get_post(intval($search_query));
if($order) {
wp_redirect(get_edit_post_link($order->ID, ''));
exit;
}
}
}
$wp_admin_bar->add_menu(array(
'id' => 'admin_bar_shop_order_form',
'title' => '<type methodology="get" motion="'.get_site_url().'/wp-admin/edit.php?post_type=shop_order">
<enter title="s" sort="textual content" placeholder="Order ID" worth="' . esc_attr($search_query) . '" type="width:100px; top: 25px; padding-left: 5px;">
<button class="button button-primary" type="top: 25px; padding: 0px 10px 0px 10px; margin-left: -5px;">Verify this order</button>
<enter title="post_type" worth="shop_order" sort="hidden">
</type>'
));
},100
);
The right way to ship a customized reminder electronic mail for WooCommerce On-Maintain orders after two days?
By default, Woocommerce doesn’t ship out reminder emails for the on-hold emails. With the assistance of this snippet right here you possibly can ship a customized reminder electronic mail for WooCommerce On-Maintain orders after two days of order completion.
NB! Take note of the road 8. In it, you possibly can change the remider delay.
add_action( 'restrict_manage_posts', 'wpsh_payment_reminder' );
operate wpsh_payment_reminder() {
international $pagenow, $post_type;
if( 'shop_order' === $post_type && 'edit.php' === $pagenow
&& get_option( 'unpaid_orders_reminder_daily_process' ) < time() ) :
$days_delay = 2; // 24 hours
$one_day = 24 * 60 * 60;
$at present = strtotime( date('Y-m-d') );
$unpaid_orders = (array) wc_get_orders( array(
'restrict' => -1,
'standing' => 'on-hold',
'date_created' => '<' . ( $at present – ($days_delay * $one_day) ),
) );
if ( sizeof($unpaid_orders) > 0 ) {
$reminder_text = __("Fee reminder electronic mail despatched to buyer $at present.", "woocommerce");
foreach ( $unpaid_orders as $order ) {
$order->update_meta_data( '_send_on_hold', true );
$order->update_status( 'reminder', $reminder_text );
$wc_emails = WC()->mailer()->get_emails(); // Get all WC_emails objects situations
$wc_emails['WC_Email_Customer_On_Hold_Order']->set off( $order->get_id() ); // Ship electronic mail
}
}
update_option( 'unpaid_orders_reminder_daily_process', $at present + $one_day );
endif;
}
add_action ( 'woocommerce_email_order_details', 'wpsh_payment_reminder_notification', 5, 4 );
operate wpsh_payment_reminder_notification( $order, $sent_to_admin, $plain_text, $electronic mail ){
if ( 'customer_on_hold_order' == $email->id && $order->get_meta('_send_on_hold') ){
$order_id = $order->get_id();
$order_link = wc_get_page_permalink('myaccount').'view-order/'.$order_id.'/';
$order_number = $order->get_order_number();
echo '<h2>'.__("Don’t forget about your order.").'</h2>
<p>'.sprintf( __("CUSTOM MESSAGE HERE… %s"),
'<a href="'.$order_link.'">'.__("Your My account order #").$order_number.'<a>'
) .'
// Ship a customized reminder electronic mail for WooCommerce On-Maintain orders after two days of order completion
add_action( 'restrict_manage_posts', 'wpsh_payment_reminder' );
operate wpsh_payment_reminder() {
international $pagenow, $post_type;
if( 'shop_order' === $post_type && 'edit.php' === $pagenow
&& get_option( 'unpaid_orders_reminder_daily_process' ) < time() ) :
$days_delay = 2; // 24 hours
$one_day = 24 * 60 * 60;
$at present = strtotime( date('Y-m-d') );
$unpaid_orders = (array) wc_get_orders( array(
'restrict' => -1,
'standing' => 'on-hold',
'date_created' => '<' . ( $at present - ($days_delay * $one_day) ),
) );
if ( sizeof($unpaid_orders) > 0 ) {
$reminder_text = __("Fee reminder electronic mail despatched to buyer $at present.", "woocommerce");
foreach ( $unpaid_orders as $order ) {
$order->update_meta_data( '_send_on_hold', true );
$order->update_status( 'reminder', $reminder_text );
$wc_emails = WC()->mailer()->get_emails(); // Get all WC_emails objects situations
$wc_emails['WC_Email_Customer_On_Hold_Order']->set off( $order->get_id() ); // Ship electronic mail
}
}
update_option( 'unpaid_orders_reminder_daily_process', $at present + $one_day );
endif;
}
add_action ( 'woocommerce_email_order_details', 'wpsh_payment_reminder_notification', 5, 4 );
operate wpsh_payment_reminder_notification( $order, $sent_to_admin, $plain_text, $electronic mail ){
if ( 'customer_on_hold_order' == $electronic mail->id && $order->get_meta('_send_on_hold') ){
$order_id = $order->get_id();
$order_link = wc_get_page_permalink('myaccount').'view-order/'.$order_id.'/';
$order_number = $order->get_order_number();
echo '<h2>'.__("Don't forget about your order.").'</h2>
<p>'.sprintf( __("CUSTOM MESSAGE HERE… %s"),
'<a href="'.$order_link.'">'.__("Your My account order #").$order_number.'<a>'
) .'</p>';
$order->delete_meta_data('_send_on_hold');
$order->save();
}
}
The right way to create customized order standing for Woocommerce?
Now let’s check out find out how to create customized order standing for Woocommerce. With the assistance of this snippet I’m going to create a customized standing referred to as “In-progress” that’s displayed on each backend and front-end order pages.
operate register_in_progress_order_status() {
register_post_status( 'wc-invoiced', array(
'label' => _x( 'In-progress', 'Order Standing', 'woocommerce' ),
'public' => true,
'exclude_from_search' => false,
'show_in_all_admin_list' => true,
'show_in_admin_status_list' => true,
'label_count' => _n_noop( 'In-progress <span class="rely">(%s)</span>', 'In-progress <span class="rely">(%s)</span>', 'woocommerce' )
)
);
}
add_action( 'init', 'register_in_progress_order_status' );
operate my_invoiced_order_status( $order_statuses ){
$order_statuses['wc-invoiced'] = _x( 'In-progress', 'Order Standing', 'woocommerce' );
return $order_statuses;
}
add_filter( 'wc_order_statuses', 'my_invoiced_order_status' );
operate show_in_bulk_actions() {
international $post_type;
if( 'shop_order' == $post_type ) {
?>
<script sort="textual content/javascript">
jQuery(doc).prepared(operate(){
jQuery('<choice>').val('mark_invoiced').textual content('<?php _e( 'Change Standing to In-progress','woocommerce' ); ?>').appendTo("choose[name='action']");
jQuery('<choice>').val('mark_invoiced').textual content('<?php _e( 'Change Standing to In-progress','woocommerce' ); ?>').appendTo("choose[name='action2']");
});
</script>
// Add customized Woocommerce order standing
operate register_in_progress_order_status() {
register_post_status( 'wc-invoiced', array(
'label' => _x( 'In-progress', 'Order Standing', 'woocommerce' ),
'public' => true,
'exclude_from_search' => false,
'show_in_all_admin_list' => true,
'show_in_admin_status_list' => true,
'label_count' => _n_noop( 'In-progress <span class="rely">(%s)</span>', 'In-progress <span class="rely">(%s)</span>', 'woocommerce' )
)
);
}
add_action( 'init', 'register_in_progress_order_status' );
operate my_invoiced_order_status( $order_statuses ){
$order_statuses['wc-invoiced'] = _x( 'In-progress', 'Order Standing', 'woocommerce' );
return $order_statuses;
}
add_filter( 'wc_order_statuses', 'my_invoiced_order_status' );
operate show_in_bulk_actions() {
international $post_type;
if( 'shop_order' == $post_type ) {
?>
<script sort="textual content/javascript">
jQuery(doc).prepared(operate(){
jQuery('<choice>').val('mark_invoiced').textual content('<?php _e( 'Change Standing to In-progress','woocommerce' ); ?>').appendTo("choose[name='action']");
jQuery('<choice>').val('mark_invoiced').textual content('<?php _e( 'Change Standing to In-progress','woocommerce' ); ?>').appendTo("choose[name='action2']");
});
</script>
<?php
}
}
add_action( 'admin_footer', 'show_in_bulk_actions' );
The right way to Filter Woocommerce orders by coupons used?
If you want to know find out how to filter Woocommerce orders by coupons used, then it’s only a snippet away. That’s, use this one right here beneath.
outlined( 'ABSPATH' ) or exit;
// fireplace it up!
add_action( 'plugins_loaded', 'wc_filter_orders_by_coupon' );
class WC_Filter_Orders_By_Coupon {
const VERSION = '1.1.0';
/** @var WC_Filter_Orders_By_Coupon single occasion of this plugin */
protected static $occasion;
public operate __construct() {
// load translations
add_action( 'init', array( $this, 'load_translation' ) );
if ( is_admin() && ! outlined( 'DOING_AJAX' ) ) {
// provides the coupon filtering dropdown to the orders web page
add_action( 'restrict_manage_posts', array( $this, 'filter_orders_by_coupon_used' ) );
// makes coupons filterable
add_filter( 'posts_join', array( $this, 'add_order_items_join' ) );
add_filter( 'posts_where', array( $this, 'add_filterable_where' ) );
}
}
public operate filter_orders_by_coupon_used() {
international $typenow;
if ( 'shop_order' === $typenow ) {
$args = array(
'posts_per_page' => – 1,
'orderby' => 'title',
'order' => 'asc',
'post_type' => 'shop_coupon',
'post_status' => 'publish',
);
$coupons = get_posts( $args );
if ( ! empty( $coupons ) ) : ?>
<choose title="_coupons_used" id="dropdown_coupons_used">
<choice worth="">
<?php esc_html_e( 'Filter by coupon used', 'wc-filter-orders' ); ?>
</choice>
<?php foreach ( $coupons as $coupon ) : ?>
<choice worth="<?php echo esc_attr( $coupon->post_title ); ?>" <?php echo esc_attr( isset( $_GET['_coupons_used'] ) ? chosen( $coupon->post_title, $_GET['_coupons_used'], false ) : '' ); ?>>
<?php echo esc_html( $coupon->post_title ); ?>
</choice>
<?php endforeach; ?>
</choose>
// Filter Woocommerce orders by coupons used
outlined( 'ABSPATH' ) or exit;
// fireplace it up!
add_action( 'plugins_loaded', 'wc_filter_orders_by_coupon' );
class WC_Filter_Orders_By_Coupon {
const VERSION = '1.1.0';
/** @var WC_Filter_Orders_By_Coupon single occasion of this plugin */
protected static $occasion;
public operate __construct() {
// load translations
add_action( 'init', array( $this, 'load_translation' ) );
if ( is_admin() && ! outlined( 'DOING_AJAX' ) ) {
// provides the coupon filtering dropdown to the orders web page
add_action( 'restrict_manage_posts', array( $this, 'filter_orders_by_coupon_used' ) );
// makes coupons filterable
add_filter( 'posts_join', array( $this, 'add_order_items_join' ) );
add_filter( 'posts_where', array( $this, 'add_filterable_where' ) );
}
}
public operate filter_orders_by_coupon_used() {
international $typenow;
if ( 'shop_order' === $typenow ) {
$args = array(
'posts_per_page' => - 1,
'orderby' => 'title',
'order' => 'asc',
'post_type' => 'shop_coupon',
'post_status' => 'publish',
);
$coupons = get_posts( $args );
if ( ! empty( $coupons ) ) : ?>
<choose title="_coupons_used" id="dropdown_coupons_used">
<choice worth="">
<?php esc_html_e( 'Filter by coupon used', 'wc-filter-orders' ); ?>
</choice>
<?php foreach ( $coupons as $coupon ) : ?>
<choice worth="<?php echo esc_attr( $coupon->post_title ); ?>" <?php echo esc_attr( isset( $_GET['_coupons_used'] ) ? chosen( $coupon->post_title, $_GET['_coupons_used'], false ) : '' ); ?>>
<?php echo esc_html( $coupon->post_title ); ?>
</choice>
<?php endforeach; ?>
</choose>
<?php endif;
}
}
public operate add_order_items_join( $be a part of ) {
international $typenow, $wpdb;
if ( 'shop_order' === $typenow && isset( $_GET['_coupons_used'] ) && ! empty( $_GET['_coupons_used'] ) ) {
$be a part of .= "LEFT JOIN {$wpdb->prefix}woocommerce_order_items woi ON {$wpdb->posts}.ID = woi.order_id";
}
return $be a part of;
}
public operate add_filterable_where( $the place ) {
international $typenow, $wpdb;
if ( 'shop_order' === $typenow && isset( $_GET['_coupons_used'] ) && ! empty( $_GET['_coupons_used'] ) ) {
// Essential WHERE question half
$the place .= $wpdb->put together( " AND woi.order_item_type='coupon' AND woi.order_item_name='%s'", wc_clean( $_GET['_coupons_used'] ) );
}
return $the place;
}
public operate load_translation() {
// localization
load_plugin_textdomain( 'wc-filter-orders', false, dirname( plugin_basename( __FILE__ ) ) . '/i18n/languages' );
}
public static operate occasion() {
if ( is_null( self::$occasion ) ) {
self::$occasion = new self();
}
return self::$occasion;
}
public operate __clone() {
/* translators: Placeholders: %s - plugin title */
_doing_it_wrong( __FUNCTION__, sprintf( esc_html__( 'You can not clone situations of %s.', 'wc-filter-orders' ), 'Filter WC Orders by Coupon' ), '1.1.0' );
}
public operate __wakeup() {
/* translators: Placeholders: %s - plugin title */
_doing_it_wrong( __FUNCTION__, sprintf( esc_html__( 'You can not unserialize situations of %s.', 'wc-filter-orders' ), 'Filter WC Orders by Coupon' ), '1.1.0' );
}
}
operate wc_filter_orders_by_coupon() {
return WC_Filter_Orders_By_Coupon::occasion();
}
The right way to filter WooCommerce orders by fee methodology?
Now let’s see find out how to filter WooCommerce orders by fee methodology. Simply use this snippet right here beneath.
outlined( 'ABSPATH' ) or exit;
// fireplace it up!
add_action( 'plugins_loaded', 'wc_filter_orders_by_payment' );
class WC_Filter_Orders_By_Payment {
const VERSION = '1.0.0';
/** @var WC_Filter_Orders_By_Payment single occasion of this plugin */
protected static $occasion;
public operate __construct() {
if ( is_admin() ) {
// add bulk order filter for exported / non-exported orders
add_action( 'restrict_manage_posts', array( $this, 'filter_orders_by_payment_method') , 20 );
add_filter( 'request', array( $this, 'filter_orders_by_payment_method_query' ) );
}
}
public operate filter_orders_by_payment_method() {
international $typenow;
if ( 'shop_order' === $typenow ) {
// get all fee strategies, even inactive ones
$gateways = WC()->payment_gateways->payment_gateways();
?>
<choose title="_shop_order_payment_method" id="dropdown_shop_order_payment_method">
<choice worth="">
<?php esc_html_e( 'All Fee Strategies', 'wc-filter-orders-by-payment' ); ?>
</choice>
<?php foreach ( $gateways as $id => $gateway ) : ?>
<choice worth="<?php echo esc_attr( $id ); ?>" <?php echo esc_attr( isset( $_GET['_shop_order_payment_method'] ) ? chosen( $id, $_GET['_shop_order_payment_method'], false ) : '' ); ?>>
<?php echo esc_html( $gateway->get_method_title() ); ?>
</choice>
<?php endforeach; ?>
</choose>
// Filter Woocommerce orders by fee methodology
outlined( 'ABSPATH' ) or exit;
// fireplace it up!
add_action( 'plugins_loaded', 'wc_filter_orders_by_payment' );
class WC_Filter_Orders_By_Payment {
const VERSION = '1.0.0';
/** @var WC_Filter_Orders_By_Payment single occasion of this plugin */
protected static $occasion;
public operate __construct() {
if ( is_admin() ) {
// add bulk order filter for exported / non-exported orders
add_action( 'restrict_manage_posts', array( $this, 'filter_orders_by_payment_method') , 20 );
add_filter( 'request', array( $this, 'filter_orders_by_payment_method_query' ) );
}
}
public operate filter_orders_by_payment_method() {
international $typenow;
if ( 'shop_order' === $typenow ) {
// get all fee strategies, even inactive ones
$gateways = WC()->payment_gateways->payment_gateways();
?>
<choose title="_shop_order_payment_method" id="dropdown_shop_order_payment_method">
<choice worth="">
<?php esc_html_e( 'All Fee Strategies', 'wc-filter-orders-by-payment' ); ?>
</choice>
<?php foreach ( $gateways as $id => $gateway ) : ?>
<choice worth="<?php echo esc_attr( $id ); ?>" <?php echo esc_attr( isset( $_GET['_shop_order_payment_method'] ) ? chosen( $id, $_GET['_shop_order_payment_method'], false ) : '' ); ?>>
<?php echo esc_html( $gateway->get_method_title() ); ?>
</choice>
<?php endforeach; ?>
</choose>
<?php
}
}
public operate filter_orders_by_payment_method_query( $vars ) {
international $typenow;
if ( 'shop_order' === $typenow && isset( $_GET['_shop_order_payment_method'] ) && ! empty( $_GET['_shop_order_payment_method'] ) ) {
$vars['meta_key'] = '_payment_method';
$vars['meta_value'] = wc_clean( $_GET['_shop_order_payment_method'] );
}
return $vars;
}
public static operate occasion() {
if ( is_null( self::$occasion ) ) {
self::$occasion = new self();
}
return self::$occasion;
}
}
operate wc_filter_orders_by_payment() {
return WC_Filter_Orders_By_Payment::occasion();
}
The right way to filter Woocommerce orders by person position?
Subsequent, let’s filter Woocommerce orders by person position. The tip result’s the one you see on the screenshot right here beneath.

operate wpsh_user_role_filter() {
international $typenow, $wp_query;
if ( in_array( $typenow, wc_get_order_types( 'order-meta-boxes' ) ) ) {
$user_role = '';
// Get all person roles
$user_roles = array( 'visitor' => 'Visitor' );
foreach ( get_editable_roles() as $key => $values ) {
$user_roles[ $key ] = $values['name'];
}
// Set a particular person position
if ( ! empty( $_GET['_user_role'] ) ) {
$user_role = sanitize_text_field( $_GET['_user_role'] );
}
// Show drop down
?><choose title='_user_role'>
<choice worth=''><?php _e( 'Choose a person position', 'woocommerce' ); ?></choice><?php
foreach ( $user_roles as $key => $worth ) :
?><choice <?php chosen( $user_role, $key ); ?> worth='<?php echo $key; ?>'><?php echo $worth; ?></choice><?php
endforeach;
?></choose>
// Filter Woocommerce orders by person position
operate wpsh_user_role_filter() {
international $typenow, $wp_query;
if ( in_array( $typenow, wc_get_order_types( 'order-meta-boxes' ) ) ) {
$user_role = '';
// Get all person roles
$user_roles = array( 'visitor' => 'Visitor' );
foreach ( get_editable_roles() as $key => $values ) {
$user_roles[ $key ] = $values['name'];
}
// Set a particular person position
if ( ! empty( $_GET['_user_role'] ) ) {
$user_role = sanitize_text_field( $_GET['_user_role'] );
}
// Show drop down
?><choose title='_user_role'>
<choice worth=''><?php _e( 'Choose a person position', 'woocommerce' ); ?></choice><?php
foreach ( $user_roles as $key => $worth ) :
?><choice <?php chosen( $user_role, $key ); ?> worth='<?php echo $key; ?>'><?php echo $worth; ?></choice><?php
endforeach;
?></choose><?php
}
}
add_action( 'restrict_manage_posts', 'wpsh_user_role_filter' );
operate wpsh_user_role_filter_where( $question ) {
if ( ! $question->is_main_query() || empty( $_GET['_user_role'] ) || $_GET['post_type'] !== 'shop_order' ) {
return;
}
if ( $_GET['_user_role'] != 'visitor' ) {
$ids = get_users( array( 'position' => sanitize_text_field( $_GET['_user_role'] ), 'fields' => 'ID' ) );
$ids = array_map( 'absint', $ids );
} else {
$ids = array( 0 );
}
$question->set( 'meta_query', array(
array(
'key' => '_customer_user',
'evaluate' => 'IN',
'worth' => $ids,
)
) );
if ( empty( $ids ) ) {
$question->set( 'posts_per_page', 0 );
}
}
add_filter( 'pre_get_posts', 'wpsh_user_role_filter_where' );
The right way to show used coupons on WooCommerce admin orders listing?
If you want to show used coupons on WooCommerce admin orders listing then use this snippet right here beneath. If no coupons is used then “No coupons used” is displayed. See the screenshot above.
add_filter( 'manage_edit-shop_order_columns', 'woo_customer_order_coupon_column_for_orders' );
operate woo_customer_order_coupon_column_for_orders( $columns ) {
$new_columns = array();
foreach ( $columns as $column_key => $column_label ) {
if ( 'order_total' === $column_key ) {
$new_columns['order_coupons'] = __('Coupons', 'woocommerce');
}
$new_columns[$column_key] = $column_label;
}
return $new_columns;
}
add_action( 'manage_shop_order_posts_custom_column' , 'woo_display_customer_order_coupon_in_column_for_orders' );
operate woo_display_customer_order_coupon_in_column_for_orders( $column ) {
international $the_order, $submit;
if( $column == 'order_coupons' ) {
if( $coupons = $the_order->get_coupon_codes() ) {
echo implode(', ', $coupons) . ' ('.rely($coupons).')';
} else {
echo '<small><em>'. __('No coupon used') . '</em>
// Show used coupons on WooCommerce admin orders listing
add_filter( 'manage_edit-shop_order_columns', 'woo_customer_order_coupon_column_for_orders' );
operate woo_customer_order_coupon_column_for_orders( $columns ) {
$new_columns = array();
foreach ( $columns as $column_key => $column_label ) {
if ( 'order_total' === $column_key ) {
$new_columns['order_coupons'] = __('Coupons', 'woocommerce');
}
$new_columns[$column_key] = $column_label;
}
return $new_columns;
}
add_action( 'manage_shop_order_posts_custom_column' , 'woo_display_customer_order_coupon_in_column_for_orders' );
operate woo_display_customer_order_coupon_in_column_for_orders( $column ) {
international $the_order, $submit;
if( $column == 'order_coupons' ) {
if( $coupons = $the_order->get_coupon_codes() ) {
echo implode(', ', $coupons) . ' ('.rely($coupons).')';
} else {
echo '<small><em>'. __('No coupon used') . '</em></small>';
}
}
}
The right way to show used coupons on Woocommerce order preview template?
With the earlier snippet we’re displaying coupons within the Woocommerce admin orders listing. Now, with the assistance of this one right here we’re going to show used coupons on Woocommerce order preview template.
add_filter( 'woocommerce_admin_order_preview_get_order_details', 'wpsh_coupon_in_order_preview', 10, 2 );
operate wpsh_coupon_in_order_preview( $information, $order ) {
// Substitute '_custom_meta_key' by the proper postmeta key
if( $coupons = $order->get_used_coupons() ) {
$information['coupons_count'] = rely($coupons); // <= Retailer the rely within the information array.
$information['coupons_codes'] = implode(', ', $coupons); // <= Retailer the rely within the information array.
}
return $information;
}
// Show coupon in Order preview
add_action( 'woocommerce_admin_order_preview_end', 'wpsh_coupon_in_order_preview_data' );
operate wpsh_coupon_in_order_preview_data(){
// Name the saved worth and show it
echo '<div><robust>' . __('Coupons used') . ' ({{information.coupons_count}})<robust>: {{information.coupons_codes}}</div>
// Show used coupons on Woocommerce order preview template
add_filter( 'woocommerce_admin_order_preview_get_order_details', 'wpsh_coupon_in_order_preview', 10, 2 );
operate wpsh_coupon_in_order_preview( $information, $order ) {
// Substitute '_custom_meta_key' by the proper postmeta key
if( $coupons = $order->get_used_coupons() ) {
$information['coupons_count'] = rely($coupons); // <= Retailer the rely within the information array.
$information['coupons_codes'] = implode(', ', $coupons); // <= Retailer the rely within the information array.
}
return $information;
}
// Show coupon in Order preview
add_action( 'woocommerce_admin_order_preview_end', 'wpsh_coupon_in_order_preview_data' );
operate wpsh_coupon_in_order_preview_data(){
// Name the saved worth and show it
echo '<div><robust>' . __('Coupons used') . ' ({{information.coupons_count}})<robust>: {{information.coupons_codes}}</div><br>';
}
The right way to show buyer telephone and electronic mail in Woocommerce orders desk?
Check out the screenshot beneath, and also you’ll see that there’s a buyer telephone and electronic mail that’s displayed in Woocommerce orders desk. If you want to realize the identical outcome, then use this snippet right here beneath.

add_action( 'manage_shop_order_posts_custom_column' , 'wpsh_phone_and_email_column', 50, 2 );
operate wpsh_phone_and_email_column( $column, $post_id ) {
if ( $column == 'order_number' )
{
international $the_order;
// Show buyer telephone in Woocommerce orders desk
if( $telephone = $the_order->get_billing_phone() ){
$phone_wp_dashicon = '<span class="dashicons dashicons-phone"></span> ';
echo '<br><a href="tel:'.$telephone.'">' . $phone_wp_dashicon . $telephone.'</a></robust>';
}
// Show buyer electronic mail in Woocommerce orders desk
if( $electronic mail = $the_order->get_billing_email() ){
echo '<br><robust><a href="mailto:'.$electronic mail.'">' . $electronic mail . '</a>
// Show buyer telephone and electronic mail in Woocommerce orders desk
add_action( 'manage_shop_order_posts_custom_column' , 'wpsh_phone_and_email_column', 50, 2 );
operate wpsh_phone_and_email_column( $column, $post_id ) {
if ( $column == 'order_number' )
{
international $the_order;
// Show buyer telephone in Woocommerce orders desk
if( $telephone = $the_order->get_billing_phone() ){
$phone_wp_dashicon = '<span class="dashicons dashicons-phone"></span> ';
echo '<br><a href="tel:'.$telephone.'">' . $phone_wp_dashicon . $telephone.'</a></robust>';
}
// Show buyer electronic mail in Woocommerce orders desk
if( $electronic mail = $the_order->get_billing_email() ){
echo '<br><robust><a href="mailto:'.$electronic mail.'">' . $electronic mail . '</a></robust>';
}
}
}
The right way to add customized button to Woocommerce orders web page (and merchandise web page)
It’s time so as to add customized button to Woocommerce orders web page (and merchandise web page). See the screenshot.

add_action( 'manage_posts_extra_tablenav', 'wpsh_button_on_orders_page', 20, 1 );
operate wpsh_button_on_orders_page( $which ) {
international $typenow;
if ( 'shop_order' === $typenow && 'prime' === $which ) {
?>
<div class="alignright actions customized">
<button sort="submit" title="custom_" type="top:32px;" class="button" worth=""><?php
// Change your URL and button textual content right here
echo __( '<a method="text-decoration: none;" href="/wp-admin/edit.php?post_type=product">
Go to merchandise »
</a>', 'woocommerce' ); ?></button>
</div>
<?php
}
}
// Add customized button to Woocommerce merchandise web page
add_action( 'manage_posts_extra_tablenav', 'wpsh_button_on_products_page', 20, 1 );
operate wpsh_button_on_products_page( $which ) {
international $typenow;
if ( 'product' === $typenow && 'prime' === $which ) {
?>
<div class="alignleft actions customized">
<button sort="submit" title="custom_" type="top:32px;" class="button" worth=""><?php
// Change your URL and button textual content right here
echo __( '<a method="text-decoration: none;" href="/wp-admin/edit.php?post_type=shop_order">
Go to orders »
</a>', 'woocommerce' ); ?></button>
</div>
// Add customized button to Woocommerce orders web page
add_action( 'manage_posts_extra_tablenav', 'wpsh_button_on_orders_page', 20, 1 );
operate wpsh_button_on_orders_page( $which ) {
international $typenow;
if ( 'shop_order' === $typenow && 'prime' === $which ) {
?>
<div class="alignright actions customized">
<button sort="submit" title="custom_" type="top:32px;" class="button" worth=""><?php
// Change your URL and button textual content right here
echo __( '<a method="text-decoration: none;" href="/wp-admin/edit.php?post_type=product">
Go to merchandise »
</a>', 'woocommerce' ); ?></button>
</div>
<?php
}
}
// Add customized button to Woocommerce merchandise web page
add_action( 'manage_posts_extra_tablenav', 'wpsh_button_on_products_page', 20, 1 );
operate wpsh_button_on_products_page( $which ) {
international $typenow;
if ( 'product' === $typenow && 'prime' === $which ) {
?>
<div class="alignleft actions customized">
<button sort="submit" title="custom_" type="top:32px;" class="button" worth=""><?php
// Change your URL and button textual content right here
echo __( '<a method="text-decoration: none;" href="/wp-admin/edit.php?post_type=shop_order">
Go to orders »
</a>', 'woocommerce' ); ?></button>
</div>
<?php
}
}
The right way to set default Woocommerce login web page to “Orders” web page?
This snippet right here beneath will redirect you to the Woocommerce “Orders” after login.
// Set default Woocommerce login web page to "Orders" web page
add_action( 'load-index.php', 'wpsh_redirect_to_orders' );
operate wpsh_redirect_to_orders(){
wp_redirect( admin_url( 'edit.php?post_type=shop_order' ) );
}
add_filter( 'login_redirect', 'wpsh_redirect_to_orders_dashboard', 9999, 3 );
operate wpsh_redirect_to_orders_dashboard( $redirect_to, $request, $person ){
$redirect_to = admin_url( 'edit.php?post_type=shop_order' );
return $redirect_to;
}
The right way to autocomplete Woocommerce processing orders?
Why would you should autocomplete Woocommerce processing orders? For instance, if you happen to’re promoting digital product (Programs and so on.) and also you want to add an entry to it proper after funds.
// Autocomplete Woocommerce processing orders
add_filter( 'woocommerce_payment_complete_order_status', 'processing_orders_autocomplete', 9999 );
operate processing_orders_autocomplete() {
return 'accomplished';
}
The right way to autocomplete all Woocommerce orders?
However (simply “however) what if you want to autocomplete all Woocommerce orders? That’s evan on-hold orders? If that is so then use this nippet right here beneath.
// Auto Full all WooCommerce orders.
add_action( 'woocommerce_thankyou', 'wpsh_complete_all_orders' );
operate wpsh_complete_all_orders( $order_id ) {
if ( ! $order_id ) {
return;
}
$order = wc_get_order( $order_id );
$order->update_status( 'accomplished' );
}
The right way to add the product picture to Woocommerce my account order view at My account web page?
What do I imply by that? Check out the screenshot beneath, and also you’ll see that there’s a picture on Woocommerce orders view at My account web page.
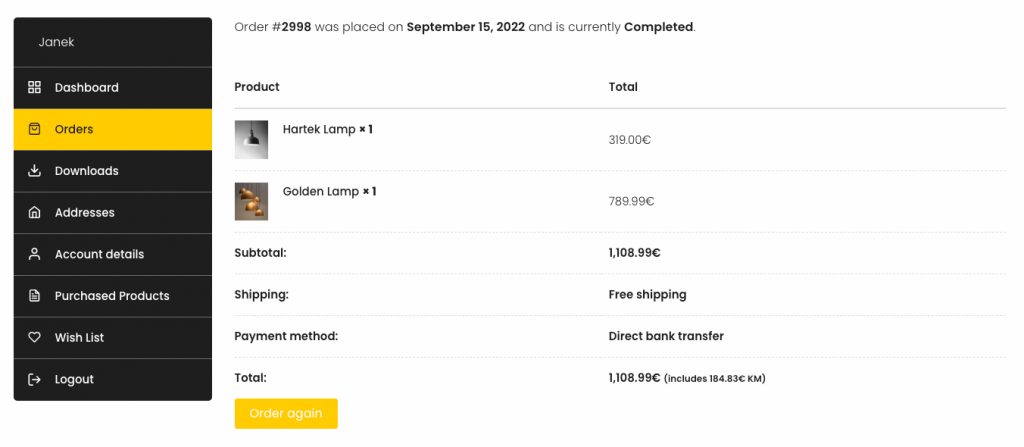
add_filter( 'woocommerce_order_item_name', 'wpsh_display_product_image_in_order_item', 20, 3 );
operate wpsh_display_product_image_in_order_item( $item_name, $merchandise, $is_visible ) {
// Focusing on view order pages solely
if( is_wc_endpoint_url( 'view-order' ) ) {
$product = $item->get_product(); // Get the WC_Product object (from order merchandise)
$thumbnail = $product->get_image(array( 50, 50)); // Get the product thumbnail (from product object)
if( $product->get_image_id() > 0 )
$item_name = '<div class="item-thumbnail">' . $thumbnail . '
// Show the product thumbnail in Woocommerce order view pages
add_filter( 'woocommerce_order_item_name', 'wpsh_display_product_image_in_order_item', 20, 3 );
operate wpsh_display_product_image_in_order_item( $item_name, $merchandise, $is_visible ) {
// Focusing on view order pages solely
if( is_wc_endpoint_url( 'view-order' ) ) {
$product = $merchandise->get_product(); // Get the WC_Product object (from order merchandise)
$thumbnail = $product->get_image(array( 50, 50)); // Get the product thumbnail (from product object)
if( $product->get_image_id() > 0 )
$item_name = '<div class="item-thumbnail">' . $thumbnail . '</div>' . $item_name;
}
return $item_name;
}