The WordPress hooks are a manner for builders to change the default conduct of the WordPress platform by “hooking” into numerous factors within the WordPress code. This permits builders so as to add their {custom} code to the platform with out having to change the core WordPress code.
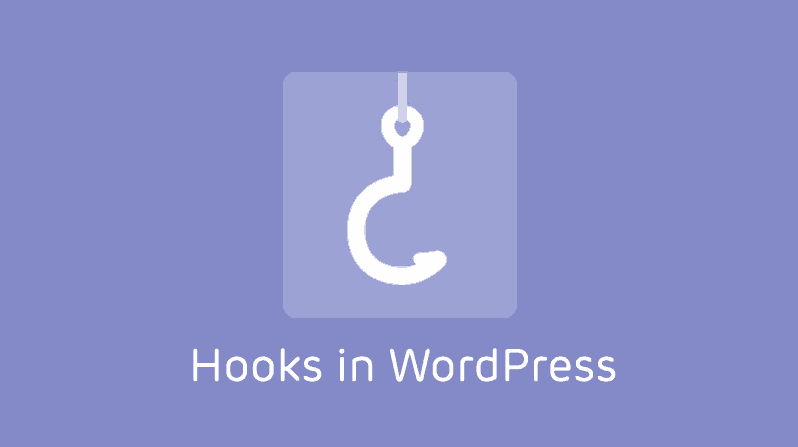
There are two varieties of hooks in WordPress: actions and filters. Actions permit builders so as to add their code at a selected level within the WordPress code, whereas filters permit builders to change knowledge earlier than it’s displayed or saved to the database.
To make use of hooks in WordPress, builders can create {custom} features after which use the add_action()
or add_filter()
features to “hook” their {custom} operate into the WordPress code.
Right here is an instance of use the add_action()
operate to create a {custom} operate that shows a message on the WordPress login web page:
operate my_login_message() { echo "Welcome to our web site! Please log in to proceed."; } add_action( 'login_form', 'my_login_message' );
On this instance, the my_login_message()
operate is the {custom} operate that may show the message. The add_action()
operate is used to “hook” the {custom} operate into the WordPress code on the login_form
motion hook. This can trigger the message to be displayed on the login type.
Right here is an instance of use the add_filter()
operate to create a {custom} operate that modifies the submit title earlier than it’s saved to the database:
operate my_modify_post_title( $title ) { $title = strtoupper( $title ); return $title; } add_filter( 'title_save_pre', 'my_modify_post_title' );
1. What are Motion hooks?
Motion hooks permit builders so as to add their code at a selected level within the WordPress code. For instance, an motion hook may be used so as to add a {custom} script to the entrance finish of the location or to ship an electronic mail when a consumer registers.
Motion hooks are triggered by particular occasions within the WordPress code, and builders can use the add_action()
operate to “hook” their {custom} operate into the WordPress code at a selected motion hook.
2. What are Filter hooks?
Filter hooks permit builders to change knowledge earlier than it’s displayed or saved to the database. For instance, a filter hook may be used to change the submit title earlier than it’s saved to the database or to change the excerpt size on archive pages.
Filter hooks are used to “filter” knowledge as it’s handed by means of the WordPress code, and builders can use the add_filter()
operate to “hook” their {custom} operate into the WordPress code at a selected filter hook.
3. The distinction between WordPress actions and filters
Each varieties of hooks can be utilized to create {custom} features that modify the default conduct of the WordPress platform.
Motion hooks permit builders so as to add {custom} code to WordPress at particular factors within the code, whereas filter hooks permit builders to change knowledge as it’s handed by means of the code.
4. Utilizing a plugin so as to add {custom} WordPress features
if you’re making a extra complicated modification that entails a number of hooks and {custom} features, it could be simpler to handle the code in a {custom} plugin.
Among the benefits embrace:
- Customized plugins could make it simpler for builders to handle and replace their {custom} code, as all the {custom} features and hooks are contained in a single plugin file. This may be particularly helpful for builders who’re engaged on a staff, because it permits them to simply share and collaborate on the {custom} code.
- Utilizing a {custom} plugin can assist to enhance the efficiency and safety of the location by making certain that the {custom} code is simply loaded when it’s wanted, slightly than being loaded on each web page of the location. This will additionally assist to scale back the chance of conflicts with different plugins or themes.
5. Examples of {custom} WordPress features utilizing hooks
These are only a few examples of the numerous {custom} features that may be created utilizing the WordPress hook system.
Including a {custom} model sheet to the entrance finish of the location:
operate my_add_custom_style() { wp_enqueue_style( 'my-custom-style', get_template_directory_uri() . '/css/{custom}.css' ); } add_action( 'wp_enqueue_scripts', 'my_add_custom_style' );
Modifying the excerpt size on archive pages:
operate my_custom_excerpt_length( $size ) { return 50; } add_filter( 'excerpt_length', 'my_custom_excerpt_length', 999 );
Including a {custom} column to the posts listing desk:
operate my_custom_columns( $columns ) { $columns['custom_field'] = __( 'Customized Area', 'textdomain' ); return $columns; } add_filter( 'manage_posts_columns', 'my_custom_columns' );
Modifying the default type order of posts on the entrance finish:
operate my_custom_sort_order( $question ) { if ( is_home() && $query->is_main_query() ) { $query->set( 'orderby', 'title' ); $query->set( 'order', 'ASC' ); } } add_action( 'pre_get_posts', 'my_custom_sort_order' );
Including a {custom} dashboard widget:
operate my_custom_dashboard_widget() { wp_add_dashboard_widget( 'custom_dashboard_widget', __( 'Customized Widget', 'textdomain' ), 'my_custom_dashboard_widget_display' ); } add_action( 'wp_dashboard_setup', 'my_custom_dashboard_widget' ); operate my_custom_dashboard_widget_display() { // Show {custom} widget content material }
Sending an electronic mail when a consumer registers:
operate my_user_register_notification( $user_id ) { $consumer = get_userdata( $user_id ); wp_mail( 'admin@instance.com', 'New Person Registered', 'A brand new consumer has registered: ' . $user->user_login ); } add_action( 'user_register', 'my_user_register_notification' );
Redirecting customers to a {custom} web page after login:
operate my_login_redirect( $redirect_to, $request, $consumer ) { if ( isset( $user->roles ) && is_array( $user->roles ) ) { if ( in_array( 'subscriber', $user->roles ) ) { return home_url( '/custom-page/' ); } else { return $redirect_to; } } } add_filter( 'login_redirect', 'my_login_redirect', 10, 3 );
Including {custom} knowledge to the consumer profile contact strategies:
operate my_custom_contact_methods( $strategies ) { $strategies['custom_field'] = 'Customized Area'; return $strategies; } add_filter( 'user_contactmethods', 'my_custom_contact_methods' );
Modifying the default deal with for WordPress emails:
operate my_custom_wp_mail_from( $from_email ) { return '{custom}@instance.com'; } add_filter( 'wp_mail_from', 'my_custom_wp_mail_from' );
Modifying the default from the identify for WordPress emails:
operate my_custom_wp_mail_from_name( $from_name ) { return 'Customized Identify'; } add_filter( 'wp_mail_from_name', 'my_custom_wp_mail_from_name' );
Modifying the default WordPress search question:
operate my_custom_search_query( $question ) { if ( $query->is_search ) { $query->set( 'post_type', array( 'submit', 'web page' ) ); } return $question; } add_filter( 'pre_get_posts', 'my_custom_search_query' );
Including a {custom} physique class to the entrance finish of the location:
operate my_custom_body_class( $courses ) { $courses[] = 'custom-class'; return $courses; } add_filter( 'body_class', 'my_custom_body_class' );
Modifying the default WordPress excerpt extra textual content:
operate my_custom_excerpt_more( $extra ) { return ' [Read more...]'; } add_filter( 'excerpt_more', 'my_custom_excerpt_more' );
Including a {custom} admin discover:
operate my_custom_admin_notice() { $display = get_current_screen(); if ( $screen->id != 'dashboard' ) { return; } ?> <div class="discover notice-info"> <p>Welcome to our web site! Please you'll want to go to the <a href="<?php echo admin_url( 'options-general.php' ); ?>">settings web page</a> to configure your web site.</p> </div> <?php } add_action( 'admin_notices', 'my_custom_admin_notice' );